명품 java 프로그래밍 5장 - Open Challenge
2022. 3. 27. 21:00ㆍJAVA
728x90
package exer;
import java.util.Scanner;
public abstract class GameObject {
protected int distance;
protected int x,y;
Game gameset = new Game();
public GameObject(int startX, int startY, int distance) {
this.x = startX;
this.y = startY;
this.distance = distance;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public boolean collide(GameObject p) {
if(this.x == p.getX() && this.y == p.getY()) {
return true;
}
else {
return false;
}
}
protected abstract void move();
protected abstract char getShape();
}
class Bear extends GameObject{
public Bear(int startX, int startY, int distance) {
super(startX, startY, distance);
}
@Override
protected void move() {
Scanner scan = new Scanner(System.in);
String direction ;
direction = scan.next();
switch(direction) {
case "s":
//아래
if(gameset.accessToMove(x+distance, y)){
x = x+distance;
}
break;
case "w":
//위
if(gameset.accessToMove(x-distance, y)){
x = x-distance;
}
break;
case "d":
//오른족
if(gameset.accessToMove(x, y+distance)){
y = y+distance;
}
break;
case "a":
//왼쪽
if(gameset.accessToMove(x, y-distance)){
y = y-distance;
}
break;
default:
return;
}
}
@Override
protected char getShape() {
char b = 'B';
return b;
}
}
class Fish extends GameObject{
public Fish(int startX, int startY, int distance) {
super(startX, startY, distance);
}
@Override
protected void move() {
Scanner scan = new Scanner(System.in);
String direction ;
int num = (int)(Math.random()*5+1);
switch(num) {
case 1:
//아래
direction = "s";
if(gameset.accessToMove(x+distance, y)) {
x = x+distance;
}
break;
case 2:
//위
direction = "w";
if(gameset.accessToMove(x-distance, y)) {
x = x-distance;
}
break;
case 3:
//오른족
direction = "d";
if(gameset.accessToMove(x, y+distance)) {
y = y+distance;
}
break;
case 4:
//왼쪽
direction = "a";
if(gameset.accessToMove(x, y-distance)) {
y = y-distance;
}
break;
default:
break;
}
}
@Override
protected char getShape() {
char f = 'F';
return f;
}
}
package exer;
public class Game {
Bear bear;
Fish fish;
// 초기화를 안해도 쓸 수 있었다..
int bear_x;
int bear_y;
int fish_x;
int fish_y;
public boolean accessToMove(int x, int y) {
if(map[x][y] == '-' || map[x][y] == 'B' || map[x][y] == 'F') {
return true;
}else {
return false;
}
}
public void gameSet() {
bear = new Bear(0,0,1);
fish = new Fish(5,6,1);
for(int i=0;i<10;i++) {
for(int j=0;j<10;j++) {
if(i == 0 && j ==0) {
map[i][j] = bear.getShape();
}
else if(i == 5 && j == 6) {
map[i][j] = fish.getShape();
}else {
map[i][j] = '-';
}
System.out.print(map[i][j]);
}
System.out.println();
}
}
char[][] map = new char[10][20];
public void printMap() {
bear_x = bear.getX();
bear_y = bear.getY();
fish_x = fish.getX();
fish_x = fish.getY();
for(int i=0;i<10;i++) {
for(int j=0;j<10;j++) {
if(i == bear_x && j == bear_y) {
map[i][j] = bear.getShape();
}
else if(i == fish_x && j == fish_y) {
map[i][j] = fish.getShape();
}else {
map[i][j] = '-';
}
System.out.print(map[i][j]);
}
System.out.println();
}
}
public void run() {
System.out.println("Bear의 Fish 먹기 게임을 시작합니다.");
gameSet();
while(!bear.collide(fish)){
bear.move();
System.out.println(bear.x);
System.out.println(bear.y);
fish.move();
System.out.println(fish.x);
System.out.println(fish.y);
printMap();
}
System.out.println("Bear Win!");
}
}
package exer;
public class GameRun {
public static void main(String[] args) {
System.out.println("Bear의 Fish 먹기 게임을 시작합니다.");
Game game = new Game();
game.run();
}
}
1. B가 안 움직임... F도 이상해(좌표이동 오류)
2. a 누르면 오류남
3. 방향키 3번 누르면 오류남
해결 해보겠습니다.
첫 번째는 map[i][j]를 출력해보니 빈칸이 나온다. map[][]이 공유가 안 되고 있는 상황 - > static map[][]으로 선언함
-- > 해결
이제보니깐 map이 이상하게 출력되고 있는 것을 확인 원래 20 x 10이여야 되는데 10 x 10으로 출력되고 있는 상황 --> 해결
좌표까지는 제대로 나왔는데 좌표자리에 F가 출력이 되지 않는다. -> x를 써야하는 자리에 y를 썼다고 한다.. -> 해결
2,3번 같은 경우에는 OutOfIndex오류이다. 이는 accessToMove메소드에서 x,y 좌표의 범위를 지정해 주지 않아서 그렇습니다. -> 해결
public boolean accessToMove(int x, int y) {
if(x >= 0 && x<20 && y>=0 && y<10) {
if(map[x][y] == '-' || map[x][y] == 'B' || map[x][y] == 'F') {
return true;
}else {
return false;
}
}else {
return false;
}
}
굳이 이렇게 수정한 이유는 후에 맵에서 이동하지 못하는 공간을 만들려고 했기 때문이다.
package exer;
import java.util.Scanner;
public abstract class GameObject {
protected int distance;
protected int x,y;
Game gameset = new Game();
public GameObject(int startX, int startY, int distance) {
this.x = startX;
this.y = startY;
this.distance = distance;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public boolean collide(GameObject p) {
if(this.x == p.getX() && this.y == p.getY()) {
return true;
}
else {
return false;
}
}
protected abstract void move();
protected abstract char getShape();
}
class Bear extends GameObject{
public Bear(int startX, int startY, int distance) {
super(startX, startY, distance);
}
@Override
protected void move() {
Scanner scan = new Scanner(System.in);
String direction ;
direction = scan.next();
switch(direction) {
case "s":
//아래
if(gameset.accessToMove(x+distance, y)){
x = x+distance;
}
break;
case "w":
//위
if(gameset.accessToMove(x-distance, y)){
x = x-distance;
}
break;
case "d":
//오른족
if(gameset.accessToMove(x, y+distance)){
y = y+distance;
}
break;
case "a":
//왼쪽
if(gameset.accessToMove(x, y-distance)){
y = y-distance;
}
break;
default:
return;
}
}
@Override
protected char getShape() {
char b = 'B';
return b;
}
}
class Fish extends GameObject{
public Fish(int startX, int startY, int distance) {
super(startX, startY, distance);
}
@Override
protected void move() {
Scanner scan = new Scanner(System.in);
String direction ;
int num = (int)(Math.random()*5+1);
switch(num) {
case 1:
//아래
direction = "s";
if(gameset.accessToMove(x+distance, y)) {
x = x+distance;
}
break;
case 2:
//위
direction = "w";
if(gameset.accessToMove(x-distance, y)) {
x = x-distance;
}
break;
case 3:
//오른족
direction = "d";
if(gameset.accessToMove(x, y+distance)) {
y = y+distance;
}
break;
case 4:
//왼쪽
direction = "a";
if(gameset.accessToMove(x, y-distance)) {
y = y-distance;
}
break;
default:
break;
}
}
@Override
protected char getShape() {
char f = 'F';
return f;
}
}
package exer;
public class Game {
Bear bear;
Fish fish;
// 초기화를 안해도 쓸 수 있었다..
int bear_x;
int bear_y;
int fish_x;
int fish_y;
public boolean accessToMove(int x, int y) {
if(x >= 0 && x<20 && y>=0 && y<10) {
if(map[x][y] == '-' || map[x][y] == 'B' || map[x][y] == 'F') {
return true;
}else {
return false;
}
}else {
return false;
}
}
public void gameSet() {
bear = new Bear(0,0,1);
fish = new Fish(5,6,1);
for(int i=0;i<10;i++) {
for(int j=0;j<20;j++) {
if(i == 0 && j ==0) {
map[i][j] = bear.getShape();
}
else if(i == 5 && j == 6) {
map[i][j] = fish.getShape();
}else {
map[i][j] = '-';
}
System.out.print(map[i][j]);
}
System.out.println();
}
}
static char[][] map = new char[10][20];
public void printMap() {
bear_x = bear.getX();
bear_y = bear.getY();
fish_x = fish.getX();
fish_y = fish.getY();
for(int i=0;i<10;i++) {
for(int j=0;j<20;j++) {
if(i == bear_x && j == bear_y) {
map[i][j] = bear.getShape();
}
else if(i == fish_x && j == fish_y) {
map[i][j] = fish.getShape();
}else {
map[i][j] = '-';
}
System.out.print(map[i][j]);
}
System.out.println();
}
}
public void run() {
System.out.println("Bear의 Fish 먹기 게임을 시작합니다.");
gameSet();
while(!bear.collide(fish)){
bear.move();
fish.move();
printMap();
}
System.out.println("Bear Win!");
}
}
package exer;
public class GameRun {
public static void main(String[] args) {
System.out.println("Bear의 Fish 먹기 게임을 시작합니다.");
Game game = new Game();
game.run();
}
}
이상입니다!!
그래도 성공했다...
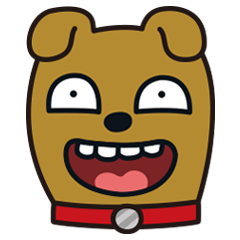
728x90
'JAVA' 카테고리의 다른 글
영어 단어 테스트 프로그램 만들기 (0) | 2022.04.03 |
---|---|
java 공부 - 명품 자바 프로그래밍 7장 11번(변형) (0) | 2022.04.02 |
java공부 - 배열에서 나타난 오류(초기화 관련) (0) | 2022.03.23 |
java- 간단한 스케줄러 만들어보기 (0) | 2022.03.18 |
java 공부 - 간단한 예약 시스템 만들기 (0) | 2022.03.18 |